Image-stitcher: A Python Implementation for Stitching Images
- Name
- Tison Brokenshire
Updated on
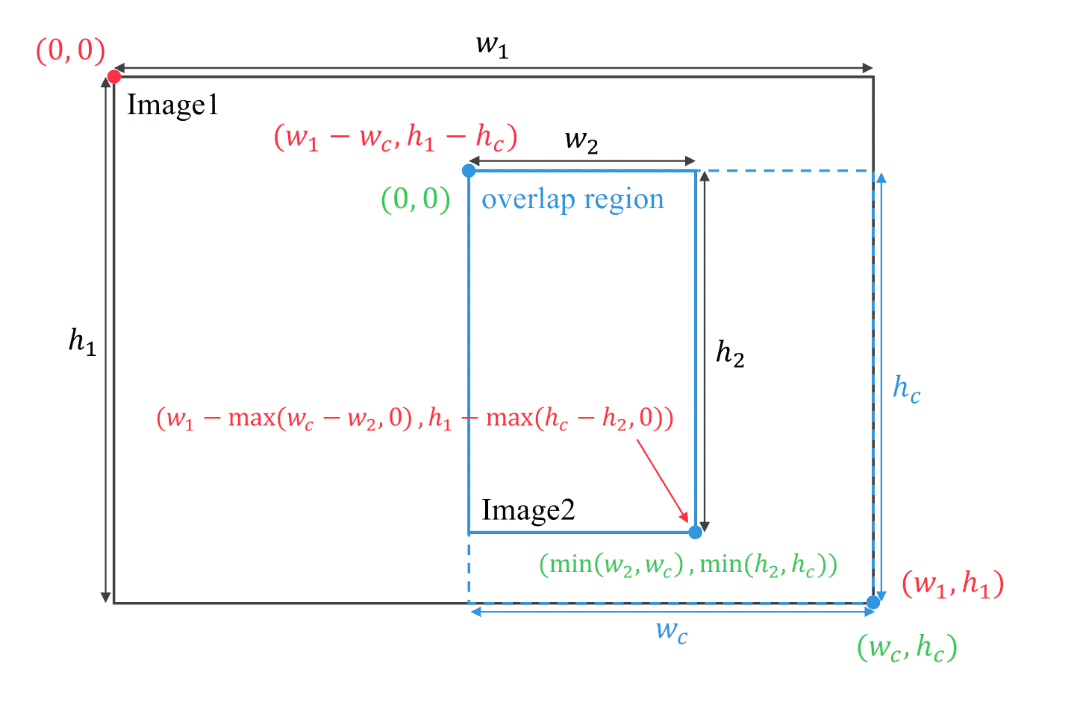
Image generation has become a pivotal part of modern digital technology, be it for creative arts, medical imaging, or panoramic views. At its core, one exciting area is the merge of multiple images into a single, coherent scene, termed image stitching. This article delves deep into an innovative Python implementation—a robust image stitcher—that promises efficiency and precision by automating the search for connecting overlaps. Buckle up as we explore the scenic path of this cutting-edge image-stitching journey!
The Concept of Image Stitching
Before plunging into the nitty-gritty of Python implementation, let's set the stage by understanding the underlying concept. Image stitching is the process of combining two or more images with overlapping fields of view to produce a high-resolution panorama or composite image. This often involves aligning features shared between images and blending them into one picture.
Practical Applications
Image stitching has myriad applications in real-world scenarios:
- Photography: Crafting stunning panoramas.
- Medical Imaging: Merging different views for better diagnostic insights.
- Satellite Imaging: Compiling large-scale maps.
- Virtual Reality: Creating immersive, 360-degree environments.
Getting Down to the Python Implementation
Our Python implementation involves a series of well-orchestrated stages: detection of key features within images, matching these features across images, estimating the transformation matrix, and finally merging the images. But here's a twist! Instead of blindly searching for overlap regions, image stitcher cleverly narrows down this search, thanks to specified overlap widths (wc) and heights (hc). This constraint significantly trims down errors and computational wastage.
Prerequisites
To get started, you'll need:
- OpenCV: For image processing and computer vision tasks.
- NumPy: For numerical operations.
- Src.main: main package.
- Src.utils.visualizer: For visualization.
Step 1: Package Import
The first step is importing main packages.
from src.main import main
from src.utils.visualizer import result_visualize
Step 2: Images Input
Just input your images by writing the code below.
merged_image, cand = main(
image1=image1, # The first image to be combined
image2=image2, # The second image to be combined
min_overlap=(5, 5), # The minimum overlap region
verbose=False, # Whether to print the log
)
Step 3: Images Merge and Visualization
The final step is visualize your result. Enter the code below and you have your images stitched.
result_visualize(
image1=image1, # The first image to be combined
image2=image2, # The second image to be combined
merged_image=merged_image, # The output image
cand=cand, # The parameters
)
The Role of Overlap Width (wc) and Height (hc)
In a conventional image stitcher, the search for overlap regions can be resource-intensive, sometimes leading to suboptimal region captures. However, by specifying the overlap region's width wc and height hc, we can limit this search space, focusing computational efforts more effectively.
Benefits
- Efficient Processing: Reduces unnecessary computations by limiting the search space.
- Improved Accuracy: Minimizes the chances of capturing incorrect overlap regions.
- Enhanced Performance: Makes the application faster and more responsive.
Putting It All Together
Here's the complete code for an efficient image stitcher in Python:
import cv2
import numpy as np
from src.main import main
from src.utils.visualizer import result_visualize
merged_image, cand = main(
image1=image1, # The first image to be combined
image2=image2, # The second image to be combined
min_overlap=(5, 5), # The minimum overlap region
verbose=False, # Whether to print the log
)
result_visualize(
image1=image1, # The first image to be combined
image2=image2, # The second image to be combined
merged_image=merged_image, # The output image
cand=cand, # The parameters
)
Wrap-up and Future Directions
Image-stitcher represents a significant advancement in image generation and merge tasks, capitalizing on Python's capabilities to deliver a smooth experience. Incorporating overlap dimensions (wc and hc) is a game-changer, ensuring both efficiency and accuracy in the stitching process.
Further enhancements could involve:
- Incorporating machine learning techniques for more robust feature detection.
- Enhancing blending algorithms to minimize visible seams.
- Extending the application to handle real-time streaming data. The world of Python-driven image processing is ever-evolving. With innovations like the image stitcher, the horizon only gets broader, offering limitless possibilities for tech enthusiasts and professionals alike.